String 객체
더보기


<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>String 객체</title>
</head>
<body>
<h2>String 객체</h2>
<script>
const str1 = '안녕하세요. JavaScript'
const str2 = new String('안녕하세요. JavaScript')
console.log(str1 == str2); // true
console.log(str1 === str2); // false
console.log(typeof(str1)); // string
console.log(typeof(str2)); // object
// length: 문자열의 길이를 반환
console.log(str1.length) // 17
// indexOf() : 특정 문자나 문장열이 처음으로 등장하는 위치를 인덱스로 반환
console.log(str1.indexOf('J')); // 7
console.log(str1.indexOf('Java')); // 7
console.log(str1.indexOf('java')); // -1 (못찾으면 -1로 표기)
console.log(str1.indexOf('a')); // 8 (처음 등장하는 위치)
console.log(str1.lastIndexOf('a')); // 10 (뒤에서 부터 찾기)
// charAt() : 특정 문자열에서 전달 받은 인덱스에 위치한 문자를 반환
console.log(str1.charAt(7)); // J
// includes() : 특정 문자열에서 전달 받은 문자열이 포함되어 있는지 여부를 반환
console.log(str1.includes('Script')) // true
console.log(str1.includes('script')) // false
// substring(): 전달 받은 시작 인덱스부터 종료 인덱스 직전까지의 문자열을 추출
console.log(str1.substring(7)); // JavaScript (7을 포함)
console.log(str1.substring(7, 11)); // Java (7포함 11직전까지)
// replace() : 원본 문자열의 일부를 전달 받은 문자열로 치환
console.log(str1.replace('안녕하세요', 'Hello')); // Hello. Javascript
// split() : 구분자를 기준으로 나눈 후 나뉜 문자열을 하나의 배열에 저장
const str3 = '김사과,오렌지,반하나,이메론,배애리,채리'
console.log(str3.split(',')); // ['김사과', '오렌지', '반하나', '이메론', '배애리', '채리']
const students = str3.split(',');
console.log(students);
for(let i in students){
console.log(i, students[i]);
}
console.log('------------------')
// 0 김사과
// 1 오렌지
// 2 반하나
// 3 이메론
// 4 배애리
// 5 채리
// trim() : 문자열의 앞 뒤 공백을 제거
const str4 = ' JavaScript '
console.log(`😀${str4}😀`); // 😀 JavaScript 😀
console.log(`😀${str4.trim()}😀`); // 😀JavaScript😀
// toUpperCase(), toLowerCase() : 문자열을 모두 대, 소문자로 변환
console.log(`😀${str4.trim().toUpperCase()}😀`); //😀JAVASCRIPT😀
</script>
</body>
</html>
Date 객체
더보기
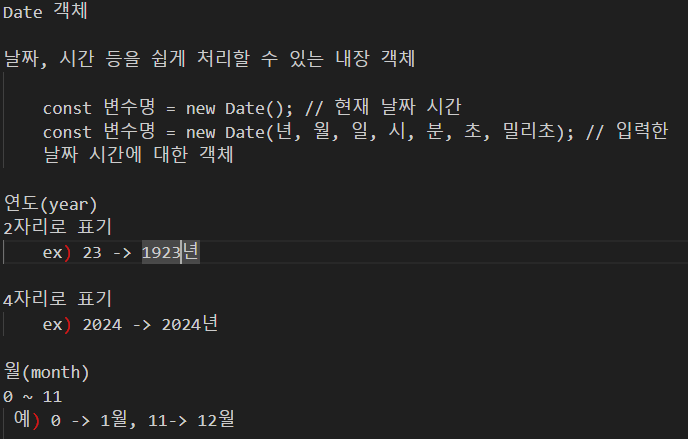

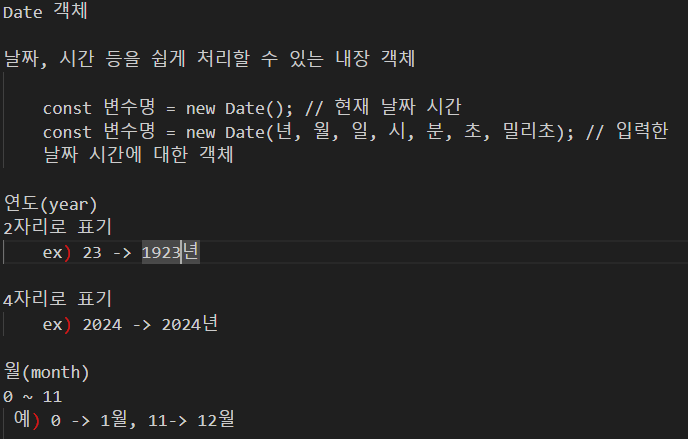
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Date 객체</title>
</head>
<body>
<h2>Date 객체</h2>
<script>
console.log(new Date()); //Wed Apr 17 2024 09:47:37 GMT+0900 (한국 표준시)
console.log(new Date(24, 10, 11)); // 1924-11-11 0시0분0초
const current_time = new Date(2024, 3, 17, 9, 51, 30, 0);
console.log(current_time);
console.log(`현재 연도: ${current_time.getFullYear()}`);
console.log(`현재 월: ${current_time.getMonth()}` );
console.log(`현재 일: ${current_time.getDate()}` );
console.log(`현재 시간: ${current_time.getHours()}` );
console.log(`현재 분: ${current_time.getMinutes()}` );
console.log(`현재 초: ${current_time.getSeconds()}` );
</script>
</body>
</html>

Form 객체
더보기
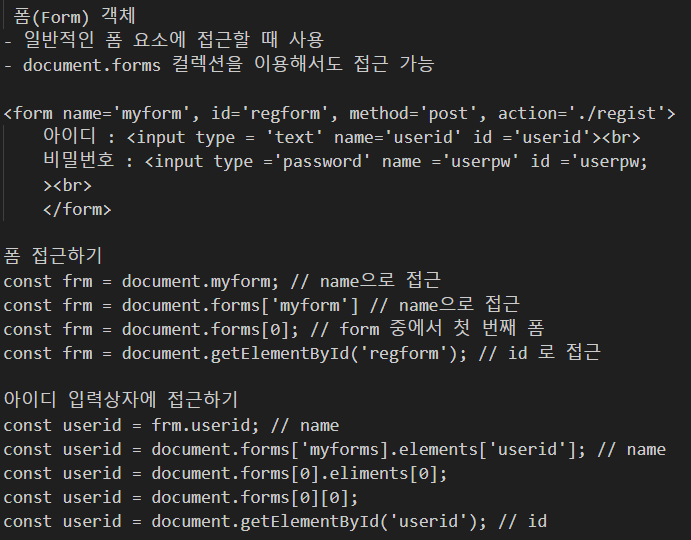

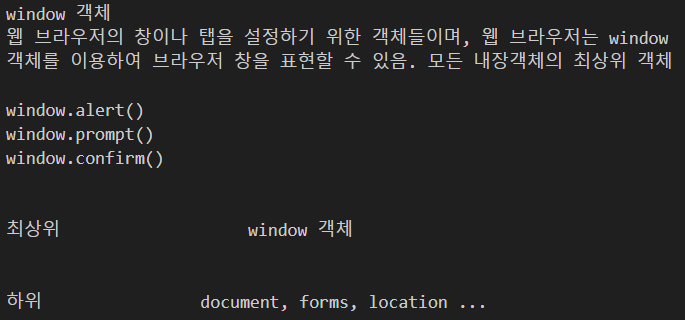
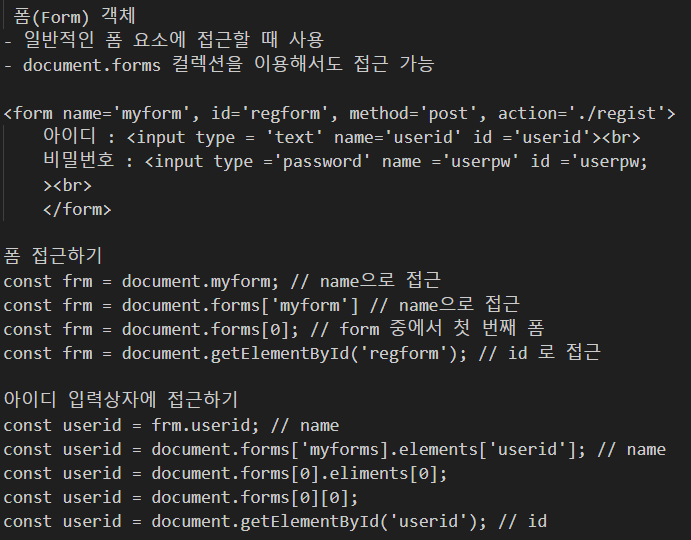
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>폼 객체</title>
</head>
<body>
<h2>폼 객체</h2>
<form action="/regist" name="frm1" id="frm1">
<input type="text" name="userid" id="userid" placeholder="아이디를입력하세요"> <br>
<input type="password" name="userpw" id="userpw" placeholder="비밀번호를 입력하세요">
</form>
<form action="/search" name="frm2" id="frm2">
<input type="search" name="search" id="search" placeholder="검색어를 입력하세요">
<button type="button" onclick="sendit()">확인</button>
</form>
<script>
// sendit은 호이스팅 되기 때문에 html보다 아래에 있어도 함수가 실행이 됨
// function형태일때는 form보다 위에 존재해도 에러가 나지 않지만
// const frm1 = document.frm1; 형태는 form보다 위에 코드를 작성하면 form을 읽어내기 전에 frm1을 호출하기 때문에 에러가 발생한다
const frm1 = document.frm1;
console.log(frm1);
console.log(frm1.userid.placeholder);
document.getElementById('userid').value = 'apple';
document.forms['frm1'].elements['userpw'].value='1004';
// frm2의 search에 있는 placeholder를 콘솔에 출력해보자
// frm2의 search를 forms 컬렉션의 인덱스로 찾아서 search 요소에 '코리아IT아카데미'를 입력
const frm2 = document.frm2;
console.log(frm2.search.placeholder);
document.forms['frm2'].elements['search'].value='코리아IT아카데미';
function sendit(){
alert('확인을 눌렀어요!')
}
</script>
</body>
</html>

++윈도우 객체
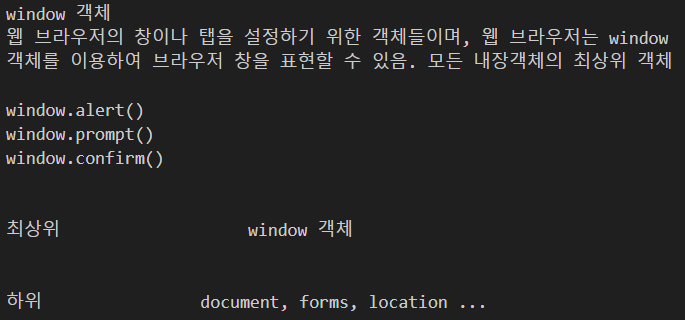
setTimeout, setInterval
더보기


<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>setTimeout</title>
</head>
<body>
<h2>setTimeout</h2>
<script>
const hello = function(){
alert('안녕하세요, JavaScript')
}
const st = setTimeout(hello, 5000); // 5초
clearTimeout(st);
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>setInterval</title>
</head>
<body>
<h2>setInterval</h2>
<script>
const hello =function(){
console.log('안녕하세요. JavaScript')
}
const si = setInterval(hello, 3000);
const clearInter = function(){
clearInterval(si);
console.log('hello()가 중지되었습니다')
}
</script>
<p><button onclick="clearInter()">중지</button></p>
</body>
</html>
문제
더보기
문제
현재 시간을 출력하는 문서를 만들어보자.
(단, 시작 버튼을 누르면 콘솔애 현재 시간을 초마다 출력하고, 중지 버튼을 누르면 시간이 정지됨. 다시 시작 버튼을 누르면 시간이 다시 출력되기 시작)
[시작][중지]
콘솔 2024-04-17 11:16:00
콘솔 2024-04-17 11:16:01
콘솔 2024-04-17 11:16:02
...
콘솔 2024-04-17 11:16:10
콘솔 2024-04-17 11:16:11
문제
현재 시간을 출력하는 문서를 만들어보자.
(단, 시작 버튼을 누르면 콘솔애 현재 시간을 초마다 출력하고, 중지 버튼을 누르면 시간이 정지됨. 다시 시작 버튼을 누르면 시간이 다시 출력되기 시작)
[시작][중지]
콘솔 2024-04-17 11:16:00
콘솔 2024-04-17 11:16:01
콘솔 2024-04-17 11:16:02
콘솔 2024-04-17 11:16:03
...
콘솔 2024-04-17 11:16:10
콘솔 2024-04-17 11:16:11
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>문제</title>
</head>
<body>
<h2>문제</h2>
<script>
function makeClock(){
const date = new Date();
yy = date.getFullYear();
MM = date.getMonth();
dd = date.getDay();
hh = date.getHours();
mm = date.getMinutes();
ss = date.getSeconds();
console.log(`${yy}-${MM}-${dd} ${hh}-${mm}-${ss}`)
}
let si;
function startClock(){
si = setInterval(makeClock, 1000);
}
function stopClock(){
clearInterval(si);
console.log('종료!');
}
</script>
<button onclick="startClock()">시작</button>
<button onclick="stopClock()">중지</button>
</body>
</html>
location, history, navigator, document 객체
더보기
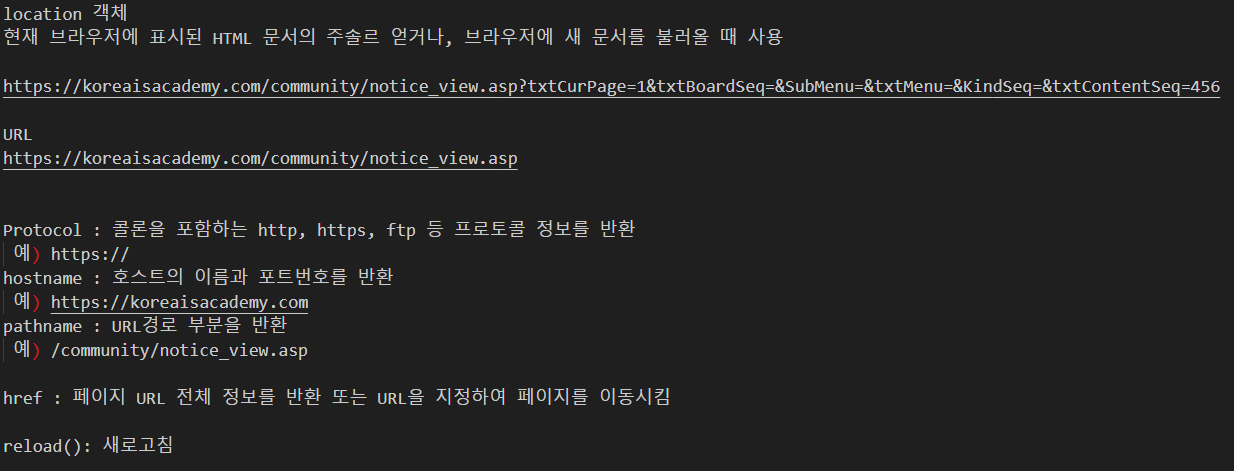







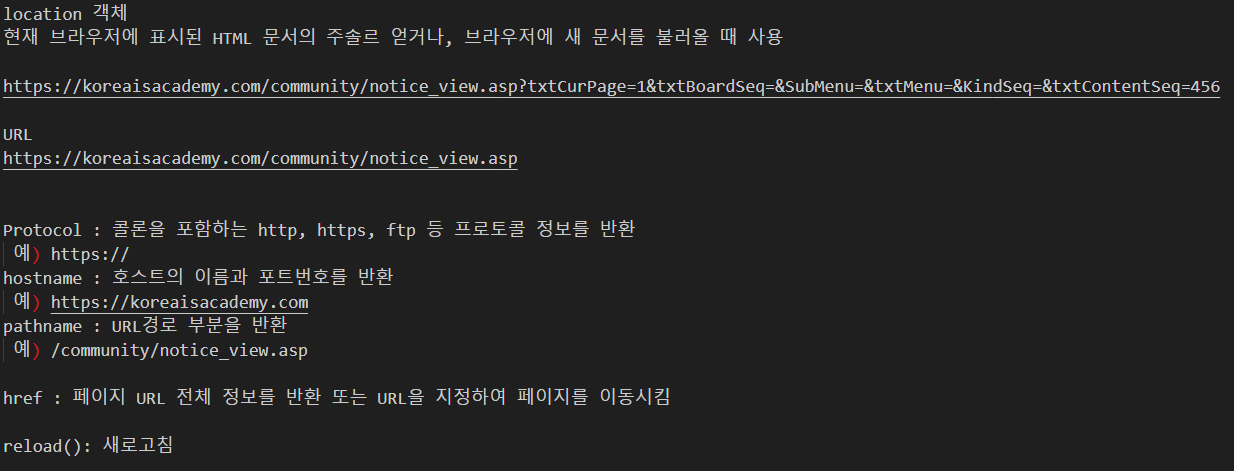
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>location 객체</title>
</head>
<body>
<h2>location 객체</h2>
<script>
function pageInfo(){
console.log(`현재 문서의 URL주소 : ${location.href}`);
console.log(`현재 문서의 protocol : ${location.protocol}`);
console.log(`현재 문서의 hostname : ${location.hostname}`);
console.log(`현재 문서의 pathname : ${location.pathname}`);
}
function sendit(){
location.href = 'https://python.org'
}
</script>
<p>
<button onclick="pageInfo()">페이지 정보</button>
<button onclick="sendit()">이동하기</button>
<button onclick="location.reload()">새로고침</button>
</p>
</body>
</html>


<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>history객체</title>
</head>
<body>
<h2>history객체</h2>
<button onclick="history.back()">뒤로</button>
<button onclick="history.forward()">앞으로</button>
<button onclick="history.go(0)">새로고침</button>
</body>
</html>


<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>navigator</title>
</head>
<body>
<h2>navigator</h2>
<script>
const success = function(loc){
console.log(loc.coords.latitude);
console.log(loc.coords.longitude);
}
const fail = function(msg){
console.log(msg.code);
}
navigator.geolocation.getCurrentPosition(success, fail)
</script>
</body>
</html>


<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>문서객체모델</title>
</head>
<body>
<h2>문서객체모델</h2>
<ul>
<li name = "markup">HTML</li>
<li>CSS</li>
<li id = "javascript" class="js">JavaScript</li>
<li class ="js">React</li>
<li class = "backend">Apache</li>
<li class = "backend">NgineX</li>
<li id ="nodejs" class = "js">Node,js</li>
<li id = "vue" class ="js">Vue</li>
</ul>
<script>
const tagName = document.getElementsByTagName('li'); // 모든 li태그를 배열로 가져옴
for(let i =0; i<tagName.length; i++){ // 배열을 for문으로 분리하고 gold색상 적용
console.log(tagName[i]);
tagName[i].style.color = 'gold';
}
const className = document.getElementsByClassName('js');
for(let i =0; i<className.length; i++){
console.log(className[i]);
className[i].style.color = 'deeppink';
}
const id = document.getElementById('javascript');
console.log(id);
id.style.color = 'greenyellow';
const name = document.getElementsByName('markup')
for(let i = 0; i <name.length; i++){
console.log(name[i])
name[i].style.color = 'deepskyblue';
}
const qsa = document.querySelectorAll('li.backend');
for(let i = 0; i <qsa.length; i++){
console.log(qsa[i])
qsa[i].style.color = 'navy';
}
</script>
</body>
</html>

<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>문서객체모델2</title>
<script>
// 윈도우에서 페이지가 로딩이 다 되면 실행해줘
window.onload = function(){
alert('문서를 다 읽었어요!')
const gnb = document.getElementById('gnb');
console.log(gnb); // nav
console.log(gnb.parentNode); // div
console.log(gnb.children[0]); // ul
console.log(gnb.children[1]); // undefined
console.log(gnb.children[0].children[0]); // li >내용1
console.log(gnb.children[0].children[0]); // li >내용1
console.log(gnb.children[0].children[0].nextElementSibling); // li > 내용2
console.log(gnb.children[0].firstChild); // #text
// firstChild -> 태그가 아니여도 가장 가까운 것 -> 띄어쓰기
// nextElementSibling -> 다음 순서 가장 가까운 태그
console.log(gnb.children[0].firstElementChild); // li > 내용1
}
</script>
</head>
<body>
<h2>문서객체모델2</h2>
<div>
<nav id="gnb">
<ul>
<li>내용 1</li>
<li>내용 2</li>
<li>내용 3</li>
</ul>
</nav>
</div>
</body>
</html>
노드(Node)
더보기
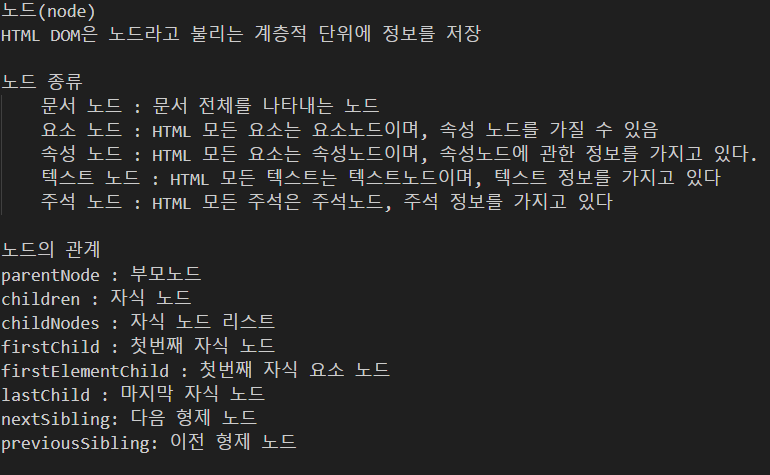
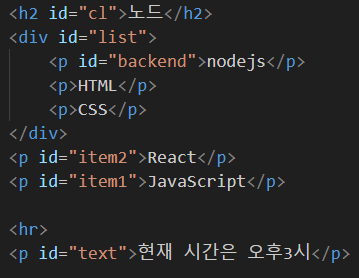









item2 -> React에 글자 색상과 배경 색상을 적용
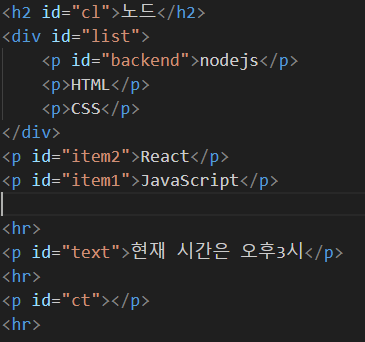

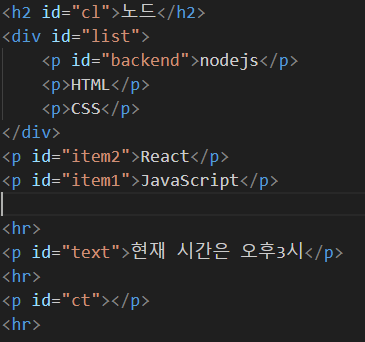
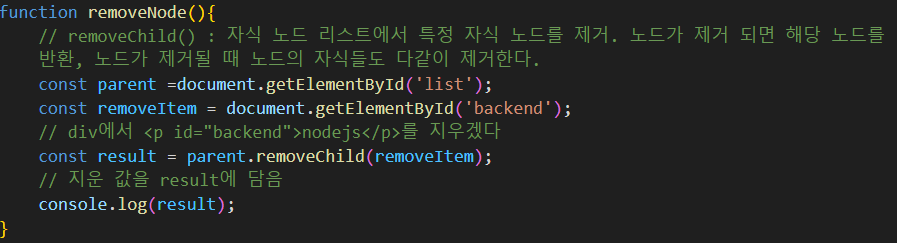


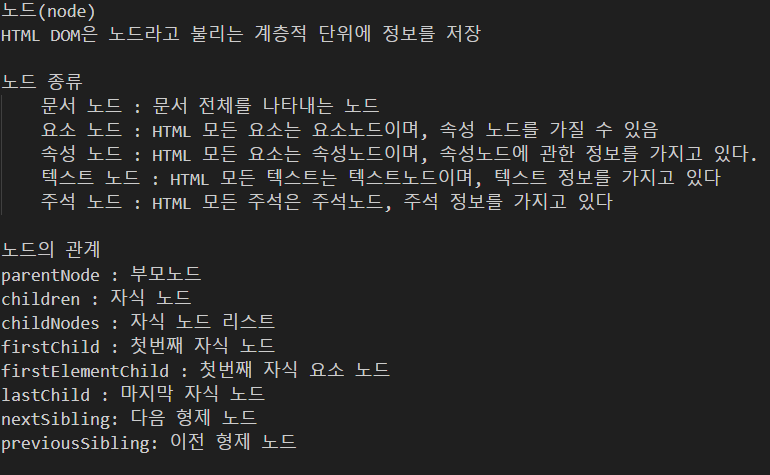
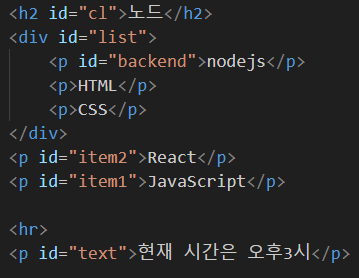



노드추가2 버튼 클릭 시 : React가 insertNode 함수의 영향으로 특정 자식 노드 앞으로 추가됨
-> React가 nodejs위로 이동


현재시간은 오후3시 - > 현재 시간은 아주 피곤한 수요일 오후3시


newItem(JavaScript) 앞에 newNode('새로운 요소가 추가됨')이 추가됨


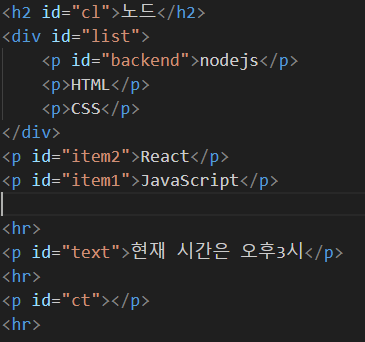

ct 가장 아래에 이모트 문장 생성
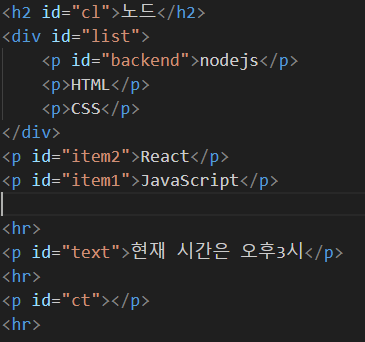
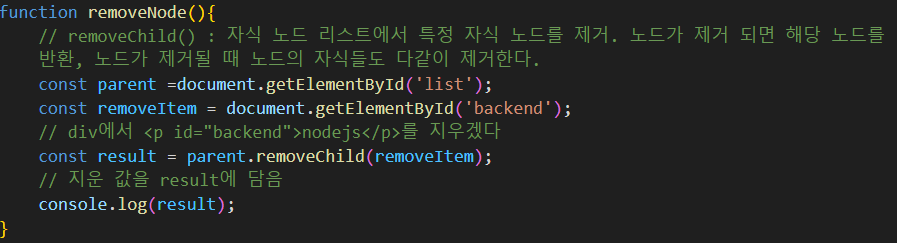


'JavaScript' 카테고리의 다른 글
정규표현식, 이벤트, 이벤트객체, 회원가입(2024-04-18) (1) | 2024.04.19 |
---|---|
프로토타입, 상속, Math, 가위바위보, 로또생성기(2024-04-16) (0) | 2024.04.16 |
while, for, break, 배열, function, 객체 (2024-04-16) (0) | 2024.04.16 |
let, const, 타입변환함수, 연산자, 대화상자 ,제어문(2024-04-15) (0) | 2024.04.15 |