더보기
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>데이터타입</title>
</head>
<body>
<h2>데이터타입</h2>
<script>
// number형
const num1 = 10;
const num2 = 11.11;
const num3 = 10e6; // 10의 6제곱
console.log(num1); // 10
console.log(num2); // 11.11
console.log(num3); // 10000000
console.log(typeof(num1)); //number
console.log(typeof(num2)); //number
console.log(typeof(num3)); //number
console.log('----------------------')
// string형
const num4 = 10;
const num5 = 5;
const str1 = 'Hello JavaScript';
const str2 = 'Hello World';
const str3 = '10';
console.log(num4 + num5); // 15
console.log(num4 + str1); // 10Hello JavaScript
console.log(str2 + str1); // Hello WorldHello JavaScript
console.log(str2 + ' ' + str1); // Hello World Hello JavaScript
console.log(`${str2} ${str1}`); // Hello World Hello JavaScript
console.log(`자바스크립트에서 문자열은 큰 따옴표 또는 작은 따옴표로 둘러싸인 문자의 집합을 의미한다`)
console.log('----------------------')
console.log(num4 + str3); // 1010 , type은 string ,숫자와 문자를 연결해줌
console.log(num4- str3); // 10 - 10 -> 0, type은 number, 유연하기 때문에 숫자와 문자를 계산할 수도 있음
console.log(num4 * str3); // 10 * 10 -> 100
console.log(num4 / str3); // 10 / 10 -> 1
console.log('----------------------')
// 논리형
const b1 = true;
const b2 = false;
console.log(typeof(b1)); // boolean
console.log(10>5); // true
console.log('----------------------')
// undefined
let num;
console.log(num); // 값 : undefined
console.log(typeof(num)); // 타입 : undefined
// null
let obj1 = {};
console.log(obj1); // {}
console.log(typeof(obj1)); // object
let obj2 = null
console.log(obj2); // null
console.log(typeof(obj2)); // object
</script>
</body>
</html>
더보기
연산자
연산자(Operator)
1. 산술연산자
+, -, *, /, %, **
2. 비교연산자
>, <, >=, <=, ==, !=, ===, !==
=== : 두 식의 값이 같고, 타입이 같아야함
3 == 3 -> true
'3' == 3 -> true
'3' === 3 -> false
!== : 두 식의 값이 다르고, 타입도 달라야 함
3. 대입연산자
=, +=, -=, *=, /=, %=, **=
4. 증감연산자
++변수, --변수, 변수++, 변수--
let num = 10
++num --> num += 1
--num --> num -= 1
num++
num--
let num = 10
result = ++num; // num =11 result =11
result = num++ // num =12 result =11
5. 논리연산자
&&(and), ||(or), !(not)
6. 비트연산자
&, |, !, ^, <<, >>
and, or, not, xor, left shift. right shift
7. 삼항연산자
변수 = 조건식 ? 반환값1 : 반환값2
- 조건식의 결과가 true 일 때 반환값1이 변수에 저장
- 조건식의 결과가 false 일 때 반환값2가 변수에 저장
더보기
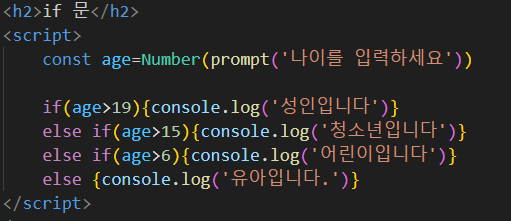
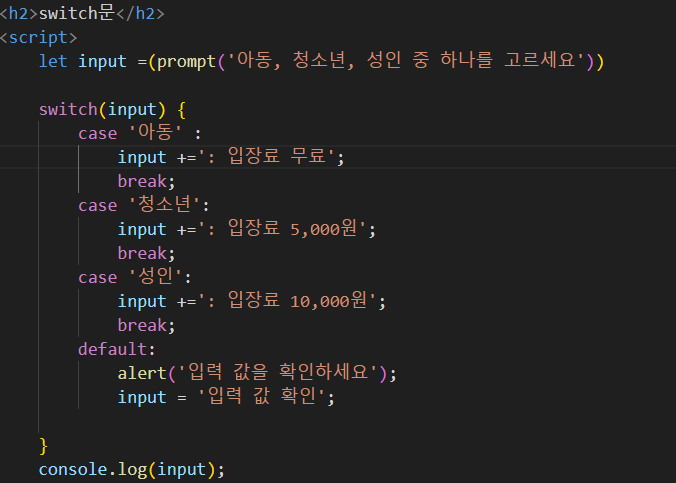
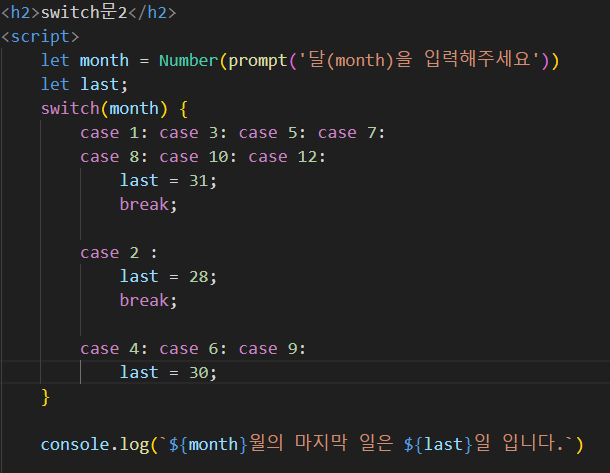
제어문
제어문
조건문
1. if 문
if(조건식){
조건식의 결과가 true일 때 실행할 문장;
}
ㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡ
if(조건식){
조건식의 결과가 true일 때 실행할 문장;
}else{
조건식의 false일 때 실행할 문장;
}
ㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡ
if(조건식1){
조건식1의 결과가 true일 때 실행할 문장;
}else if(조건식2){
조건식2의 결과가 true일 때 실행할 문장;
}else{
모든 조건식의 결과가 false일 때 실행할 문장;}
2. switch 문
switch(변수 또는 값){
case 값1 :
변수와 값1이 같은 경우 실행할 문장;
break;
case 값2 :
변수와 값2가 같은 경우 실행할 문장;
break;
...
default:
변수와 모든 값이 다를 경우 실행할 문장;
}
문제.
달(month)을 입력받아 해당 달의 마지막 일이 며칠인지 출력하는 문서를 만들어보자.
(단, 입력은 prompt로 받으며, 2월의 마지막 날짜는 28일로 함)
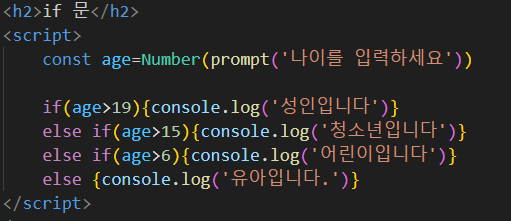
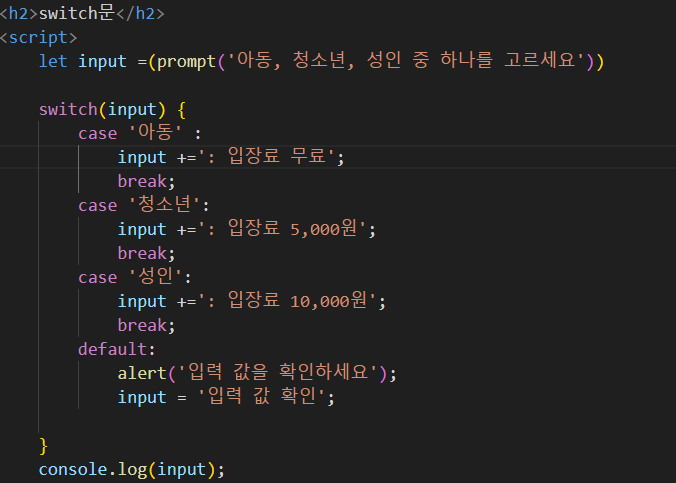
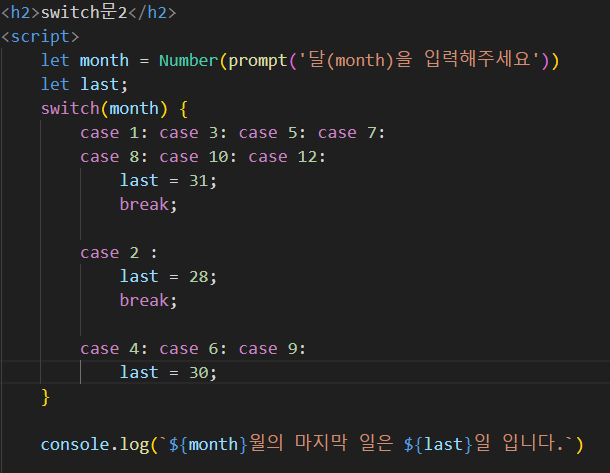
'JavaScript' 카테고리의 다른 글
정규표현식, 이벤트, 이벤트객체, 회원가입(2024-04-18) (1) | 2024.04.19 |
---|---|
string, date, form, setTimeout, setInterval(2024-04-17) (1) | 2024.04.18 |
프로토타입, 상속, Math, 가위바위보, 로또생성기(2024-04-16) (0) | 2024.04.16 |
while, for, break, 배열, function, 객체 (2024-04-16) (0) | 2024.04.16 |